

To make it even more interesting, there doesn't seem to be very good in-built support for finding sub-arrays in Numpy, needed to identify your streaks. So your loops: for experimentNumber in range(n):
Head and tails coin flip code#
Code that uses it properly looks somewhat alien to the way we natively think about numerical operations: all of the loops are hidden away in library calls, and this process is called vectorisation. Numpy is a library written in C to make fast(er) numerical operations accessible to Python. Python is slow, and the only practical way to make it fast is to not use it (at least for CPU-bound problems). The following is meant to be an interest pique and not necessarily criticism, since it's possible that you haven't seen numerical code yet. Print('Chance of streak: %s%%' % (numberOfStreaks / 100)) # find out what percentage contains a streak of six heads or six tails in a row # resets the results list for the next expirementNumber # breaks the loop after a streak has come up # takes the string down to 0 after has hit 6 # if statement for when the streak reaches 6 # else statement for when it's not the first flip or a repeating value # if the values are the same, the streak increases # checks if the current indexed value is equal to the indexed value before it # else if statement to check for repeating values # augmented assignment operator used for consistency # if statement for the first flip to start the streak # for loop where the range is equal to the length of the results variable # second part of the experiment- checks flip results for a streak # using the append method to add flip outcome to the results variable # for loop that specifies a range of 100 flips
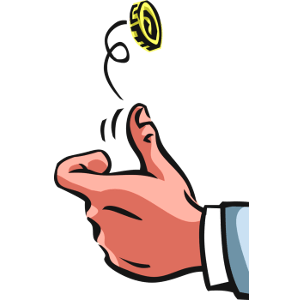
# first part of the experiment- 100 coin flips using randint # creating a loop that repeats the experiment 10,000 times # creating variables for the number of streaks, current streak and coin flip results # importing the randint function from the random module Put all of this code in a loop that repeats the experiment 10,000 times so we can find out what percentage of the coin flips contains a streak of six heads or tails in a row. Your program breaks up the experiment into two parts: the first part generates a list of randomly selected 'heads' and 'tails' values, and the second part checks if there is a streak in it. Write a program to find out how often a streak of six heads or a streak of six tails comes up in a randomly generated list of heads and tails. I'm a beginner and have only included code that I have learned up to this point in the book. With that assumption in mind, I would appreciate any feedback on my solution and comments to the problem posted below. The bold text leads me to believe that I should be checking for a streak and not multiple streaks within each experiment of 100 flips. Below is the wording from the problem in Automate the Boring Stuff book.
